集成 Socket.IO
¥Integrating Socket.IO
Socket.IO 由两部分组成:
¥Socket.IO is composed of two parts:
与 Node.JS HTTP Server(
socket.io
包)集成(或安装)的服务器¥A server that integrates with (or mounts on) the Node.JS HTTP Server (the
socket.io
package)在浏览器端加载的客户端库(
socket.io-client
包)¥A client library that loads on the browser side (the
socket.io-client
package)
在开发过程中,socket.io
自动为我们服务客户端,正如我们将看到的,所以现在我们只需要安装一个模块:
¥During development, socket.io
serves the client automatically for us, as we’ll see, so for now we only have to install one module:
npm install socket.io
这将安装该模块并将依赖添加到 package.json
。现在让我们编辑 index.js
添加它:
¥That will install the module and add the dependency to package.json
. Now let’s edit index.js
to add it:
- CommonJS
- ES modules
const express = require('express');
const { createServer } = require('node:http');
const { join } = require('node:path');
const { Server } = require('socket.io');
const app = express();
const server = createServer(app);
const io = new Server(server);
app.get('/', (req, res) => {
res.sendFile(join(__dirname, 'index.html'));
});
io.on('connection', (socket) => {
console.log('a user connected');
});
server.listen(3000, () => {
console.log('server running at http://localhost:3000');
});
import express from 'express';
import { createServer } from 'node:http';
import { fileURLToPath } from 'node:url';
import { dirname, join } from 'node:path';
import { Server } from 'socket.io';
const app = express();
const server = createServer(app);
const io = new Server(server);
const __dirname = dirname(fileURLToPath(import.meta.url));
app.get('/', (req, res) => {
res.sendFile(join(__dirname, 'index.html'));
});
io.on('connection', (socket) => {
console.log('a user connected');
});
server.listen(3000, () => {
console.log('server running at http://localhost:3000');
});
请注意,我通过传递 server
(HTTP 服务器)对象来初始化 socket.io
的新实例。然后,我监听传入套接字的 connection
事件并将其记录到控制台。
¥Notice that I initialize a new instance of socket.io
by passing the server
(the HTTP server) object. Then I listen on the connection
event for incoming sockets and log it to the console.
现在在 index.html 中的 </body>
(结束正文标记)之前添加以下代码片段:
¥Now in index.html add the following snippet before the </body>
(end body tag):
- ES6
- ES5
<script src="/socket.io/socket.io.js"></script>
<script>
const socket = io();
</script>
<script src="/socket.io/socket.io.js"></script>
<script>
var socket = io();
</script>
这就是加载 socket.io-client
所需的全部操作,它公开了 io
全局变量(和端点 GET /socket.io/socket.io.js
),然后进行连接。
¥That’s all it takes to load the socket.io-client
, which exposes an io
global (and the endpoint GET /socket.io/socket.io.js
), and then connect.
如果你想使用本地版本的客户端 JS 文件,可以在 node_modules/socket.io/client-dist/socket.io.js
找到它。
¥If you would like to use the local version of the client-side JS file, you can find it at node_modules/socket.io/client-dist/socket.io.js
.
你还可以使用 CDN 代替本地文件(例如 <script src="https://cdn.socket.io/4.8.1/socket.io.min.js"></script>
)。
¥You can also use a CDN instead of the local files (e.g. <script src="https://cdn.socket.io/4.8.1/socket.io.min.js"></script>
).
请注意,当我调用 io()
时,我没有指定任何 URL,因为它默认尝试连接到提供该页面的主机。
¥Notice that I’m not specifying any URL when I call io()
, since it defaults to trying to connect to the host that serves the page.
如果你使用反向代理(例如 apache 或 nginx),请查看 它的文档。
¥If you're behind a reverse proxy such as apache or nginx please take a look at the documentation for it.
如果你将应用托管在不是网站根目录的文件夹中(例如 https://example.com/chatapp
),那么你还需要在服务器和客户端中指定 路径。
¥If you're hosting your app in a folder that is not the root of your website (e.g., https://example.com/chatapp
) then you also need to specify the path in both the server and the client.
如果你现在重新启动该过程(通过按 Control+C 并再次运行 node index.js
),然后刷新网页,你应该会看到控制台打印“用户已连接”。
¥If you now restart the process (by hitting Control+C and running node index.js
again) and then refresh the webpage you should see the console print “a user connected”.
尝试打开多个选项卡,你会看到几条消息。
¥Try opening several tabs, and you’ll see several messages.
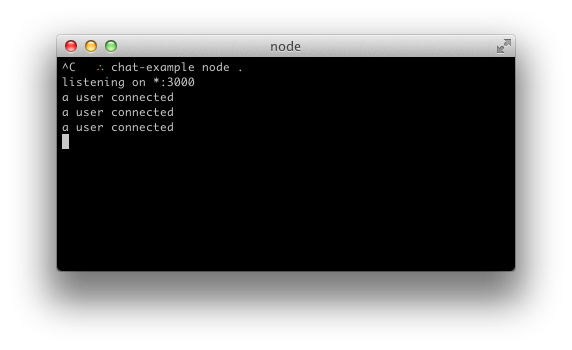
每个套接字还会触发一个特殊的 disconnect
事件:
¥Each socket also fires a special disconnect
event:
io.on('connection', (socket) => {
console.log('a user connected');
socket.on('disconnect', () => {
console.log('user disconnected');
});
});
然后,如果你多次刷新选项卡,你就可以看到它的运行情况。
¥Then if you refresh a tab several times you can see it in action.
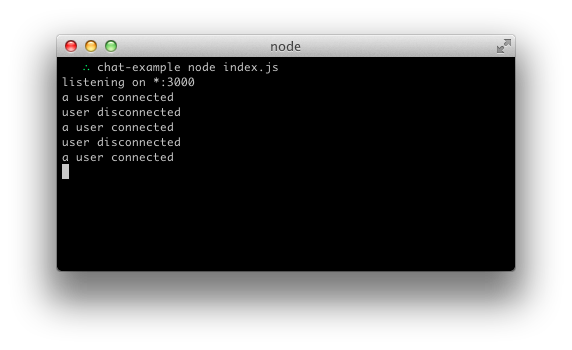
- CommonJS
- ES modules