MongoDB 适配器
工作原理
¥How it works
MongoDB 适配器依赖于 MongoDB 的 改变流(因此需要副本集或分片集群)。
¥The MongoDB adapter relies on MongoDB's Change Streams (and thus requires a replica set or a sharded cluster).
发送到多个客户端(例如 io.to("room1").emit()
或 socket.broadcast.emit()
)的每个数据包是:
¥Every packet that is sent to multiple clients (e.g. io.to("room1").emit()
or socket.broadcast.emit()
) is:
发送到连接到当前服务器的所有匹配客户端
¥sent to all matching clients connected to the current server
插入到 MongoDB capped 集合中,并由集群中的其他 Socket.IO 服务器接收
¥inserted in a MongoDB capped collection, and received by the other Socket.IO servers of the cluster
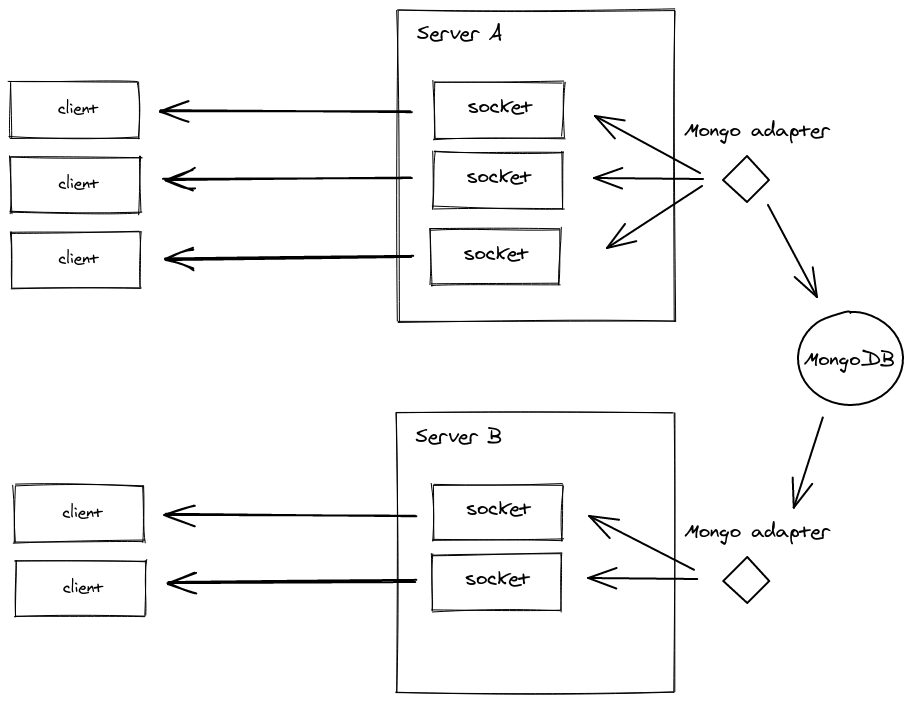
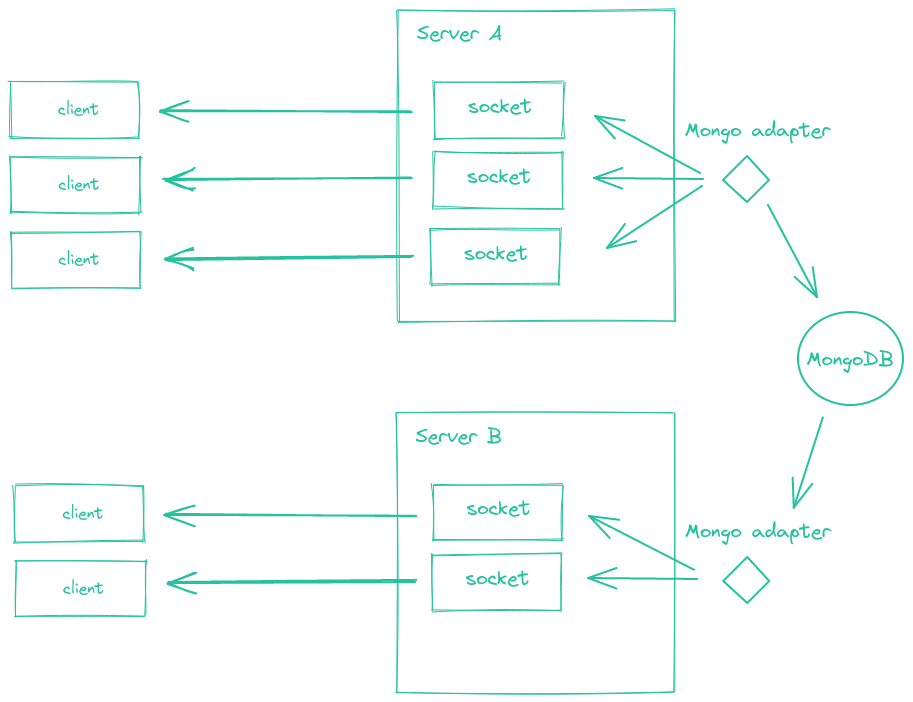
该适配器的源代码可以找到 此处。
¥The source code of this adapter can be found here.
支持的功能
¥Supported features
特性 | socket.io 版本 | 支持 |
---|---|---|
套接字管理 | 4.0.0 | :白色复选标记:是(自版本 0.1.0 起) |
服务器间通信 | 4.1.0 | :白色复选标记:是(自版本 0.1.0 起) |
广播并致谢 | 4.5.0 | :白色复选标记:是(自版本 0.2.0 起) |
连接状态恢复 | 4.6.0 | :白色复选标记:是(自版本 0.3.0 起) |
安装
¥Installation
npm install @socket.io/mongo-adapter mongodb
对于 TypeScript 用户,你可能还需要 @types/mongodb
。
¥For TypeScript users, you might also need @types/mongodb
.
用法
¥Usage
Socket.IO 集群内的广播数据包是通过创建 MongoDB 文档并在每个 Socket.IO 服务器上使用 改变流 来实现的。
¥Broadcasting packets within a Socket.IO cluster is achieved by creating MongoDB documents and using a change stream on each Socket.IO server.
清理 MongoDB 中的文档有两种方法:
¥There are two ways to clean up the documents in MongoDB:
与上限集合一起使用
¥Usage with a capped collection
import { Server } from "socket.io";
import { createAdapter } from "@socket.io/mongo-adapter";
import { MongoClient } from "mongodb";
const DB = "mydb";
const COLLECTION = "socket.io-adapter-events";
const io = new Server();
const mongoClient = new MongoClient("mongodb://localhost:27017/?replicaSet=rs0");
await mongoClient.connect();
try {
await mongoClient.db(DB).createCollection(COLLECTION, {
capped: true,
size: 1e6
});
} catch (e) {
// collection already exists
}
const mongoCollection = mongoClient.db(DB).collection(COLLECTION);
io.adapter(createAdapter(mongoCollection));
io.listen(3000);
与 TTL 索引一起使用
¥Usage with a TTL index
import { Server } from "socket.io";
import { createAdapter } from "@socket.io/mongo-adapter";
import { MongoClient } from "mongodb";
const DB = "mydb";
const COLLECTION = "socket.io-adapter-events";
const io = new Server();
const mongoClient = new MongoClient("mongodb://localhost:27017/?replicaSet=rs0");
await mongoClient.connect();
const mongoCollection = mongoClient.db(DB).collection(COLLECTION);
await mongoCollection.createIndex(
{ createdAt: 1 },
{ expireAfterSeconds: 3600, background: true }
);
io.adapter(createAdapter(mongoCollection, {
addCreatedAtField: true
}));
io.listen(3000);
选项
¥Options
名称 | 描述 | 默认值 | 添加于 |
---|---|---|---|
uid | 该节点的 ID | 随机 ID | v0.1.0 |
requestsTimeout | 服务器间请求(例如带有 ack 的 fetchSockets() 或 serverSideEmit() )的超时 | 5000 | v0.1.0 |
heartbeatInterval | 两次心跳之间的毫秒数 | 5000 | v0.1.0 |
heartbeatTimeout | 在我们考虑节点关闭之前没有心跳的毫秒数 | 10000 | v0.1.0 |
addCreatedAtField | 是否为每个 MongoDB 文档添加 createdAt 字段 | false | v0.2.0 |
常见问题
¥Common questions
使用 MongoDB 适配器时是否仍需要启用粘性会话?
¥Do I still need to enable sticky sessions when using the MongoDB adapter?
是的。如果不这样做,将导致 HTTP 400 响应(你正在访问不知道 Socket.IO 会话的服务器)。
¥Yes. Failing to do so will result in HTTP 400 responses (you are reaching a server that is not aware of the Socket.IO session).
更多信息可参见 此处。
¥More information can be found here.
当 MongoDB 集群宕机时会发生什么?
¥What happens when the MongoDB cluster is down?
如果与 MongoDB 集群的连接被切断,行为将取决于 MongoDB 客户端的 bufferMaxEntries
选项的值:
¥In case the connection to the MongoDB cluster is severed, the behavior will depend on the value of the bufferMaxEntries
option of the MongoDB client:
如果其值为
-1
(默认),则数据包将被缓冲,直到重新连接。¥if its value is
-1
(default), the packets will be buffered until reconnection.如果它的值为
0
,则数据包只会发送到连接到当前服务器的客户端。¥if its value is
0
, the packets will only be sent to the clients that are connected to the current server.
文档:http://mongodb.github.io/node-mongodb-native/3.6/api/global.html#MongoClientOptions
¥Documentation: http://mongodb.github.io/node-mongodb-native/3.6/api/global.html#MongoClientOptions
最新版本
¥Latest releases
版本 | 发布日期 | 发行说明 | 差异 |
---|---|---|---|
0.3.2 | 2024 年 1 月 | link | 0.3.1...0.3.2 |
0.3.1 | 2024 年 1 月 | link | 0.3.0...0.3.1 |
0.3.0 | 2023 年 2 月 | link | 0.2.1...0.3.0 |
0.2.1 | 2022 年 5 月 | link | 0.2.0...0.2.1 |
0.2.0 | 2022 年 4 月 | link | 0.1.0...0.2.0 |
0.1.0 | 2021 年 6 月 | link |
触发器
¥Emitter
MongoDB 触发器允许从另一个 Node.js 进程向连接的客户端发送数据包:
¥The MongoDB emitter allows sending packets to the connected clients from another Node.js process:
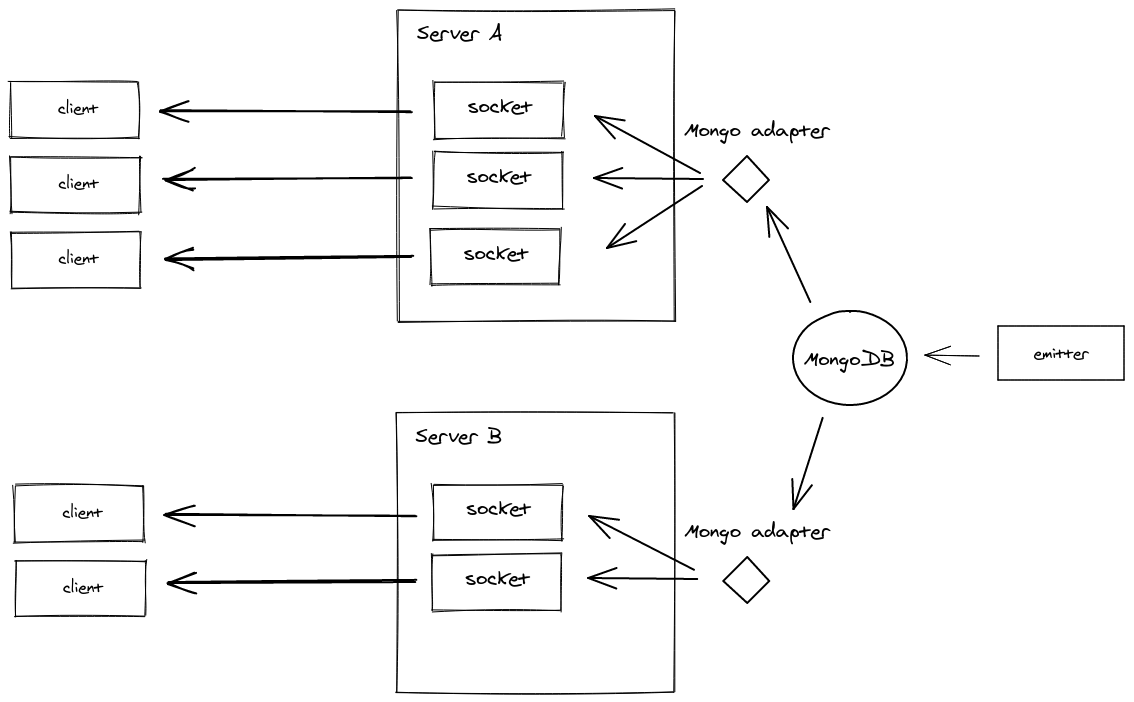
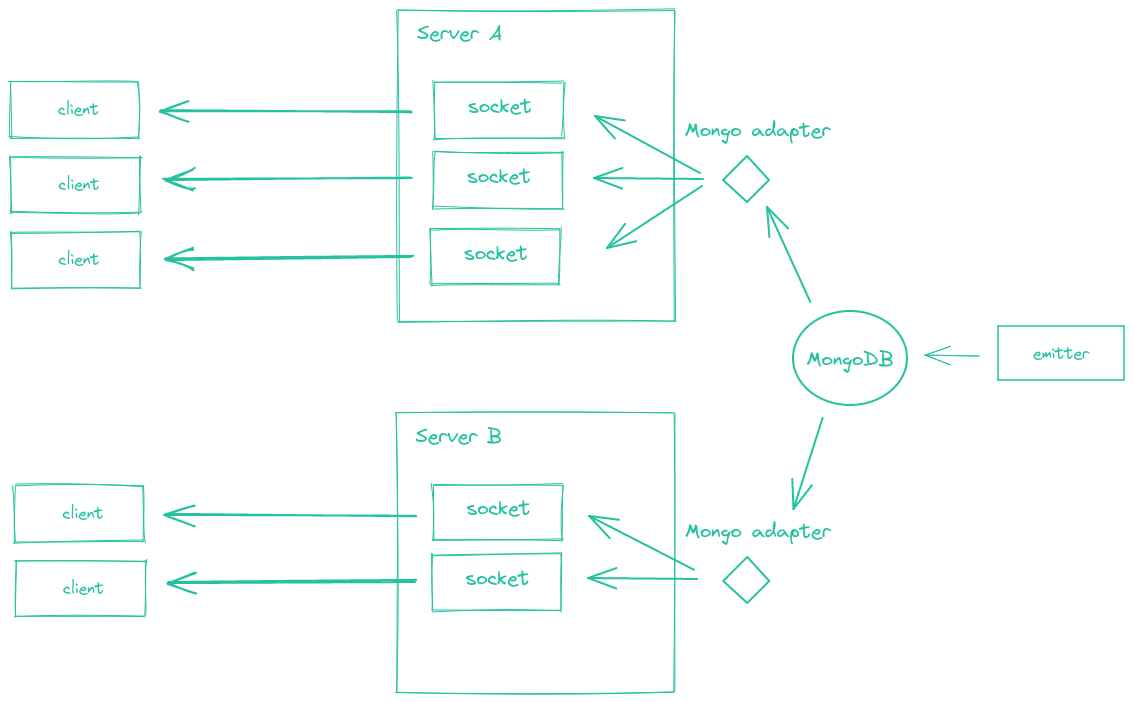
安装
¥Installation
npm install @socket.io/mongo-emitter mongodb
用法
¥Usage
const { Emitter } = require("@socket.io/mongo-emitter");
const { MongoClient } = require("mongodb");
const mongoClient = new MongoClient("mongodb://localhost:27017/?replicaSet=rs0");
const main = async () => {
await mongoClient.connect();
const mongoCollection = mongoClient.db("mydb").collection("socket.io-adapter-events");
const emitter = new Emitter(mongoCollection);
setInterval(() => {
emitter.emit("ping", new Date());
}, 1000);
}
main();
请参阅备忘单 此处。
¥Please refer to the cheatsheet here.