广播事件
Socket.IO 可以轻松地将事件发送到所有连接的客户端。
¥Socket.IO makes it easy to send events to all the connected clients.
请注意,广播是仅服务器功能。
¥Please note that broadcasting is a server-only feature.
致所有已连接的客户端
¥To all connected clients
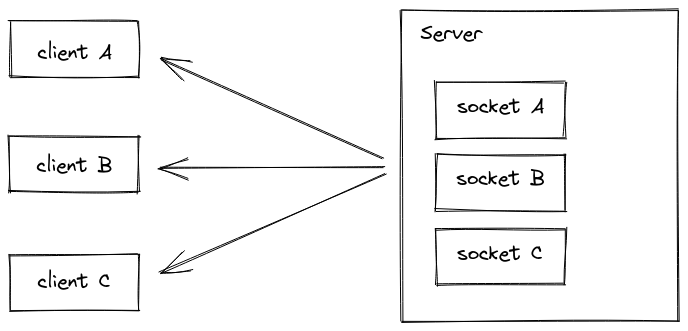
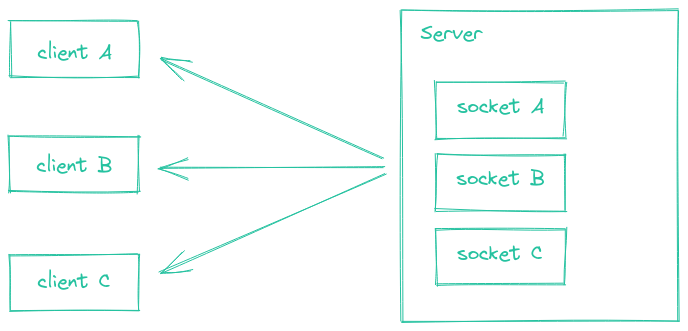
io.emit("hello", "world");
当前断开连接(或正在重新连接的过程中)的客户端将不会收到该事件。将此事件存储在某个地方(例如,在数据库中)由你决定,具体取决于你的用例。
¥Clients that are currently disconnected (or in the process of reconnecting) won't receive the event. Storing this event somewhere (in a database, for example) is up to you, depending on your use case.
至除发送者之外的所有已连接客户端
¥To all connected clients except the sender
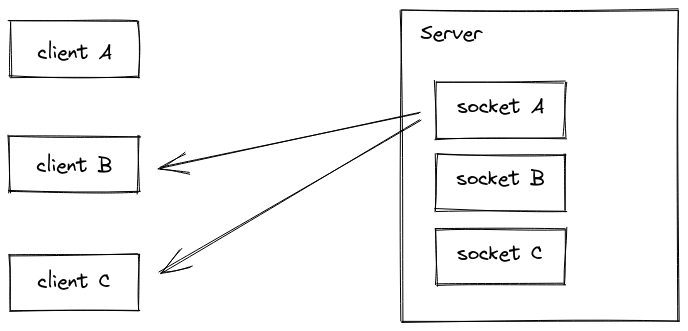
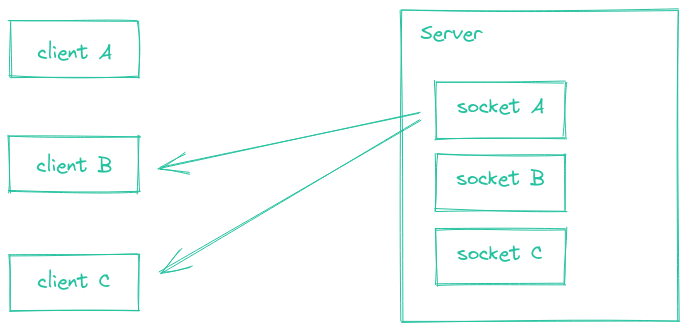
io.on("connection", (socket) => {
socket.broadcast.emit("hello", "world");
});
在上面的示例中,使用 socket.emit("hello", "world")
(没有 broadcast
标志)会将事件发送到 "客户端 A"。你可以在 cheatsheet.txt 中找到发送事件的所有方式的列表。
¥In the example above, using socket.emit("hello", "world")
(without broadcast
flag) would send the event to "client A". You can find the list of all the ways to send an event in the cheatsheet.
使用回执
¥With acknowledgements
从 Socket.IO 4.5.0 开始,你现在可以向多个客户端广播一个事件并期待每个客户端的确认:
¥Starting with Socket.IO 4.5.0, you can now broadcast an event to multiple clients and expect an acknowledgement from each one of them:
io.timeout(5000).emit("hello", "world", (err, responses) => {
if (err) {
// some clients did not acknowledge the event in the given delay
} else {
console.log(responses); // one response per client
}
});
支持所有广播形式:
¥All broadcasting forms are supported:
在一个房间里
¥in a room
io.to("room123").timeout(5000).emit("hello", "world", (err, responses) => {
// ...
});
从特定的
socket
¥from a specific
socket
socket.broadcast.timeout(5000).emit("hello", "world", (err, responses) => {
// ...
});
在命名空间中
¥in a namespace
io.of("/the-namespace").timeout(5000).emit("hello", "world", (err, responses) => {
// ...
});
使用多个 Socket.IO 服务器
¥With multiple Socket.IO servers
广播也适用于多个 Socket.IO 服务器。
¥Broadcasting also works with multiple Socket.IO servers.
你只需将默认适配器替换为 Redis 适配器 或另一个 兼容适配器。
¥You just need to replace the default adapter by the Redis Adapter or another compatible adapter.
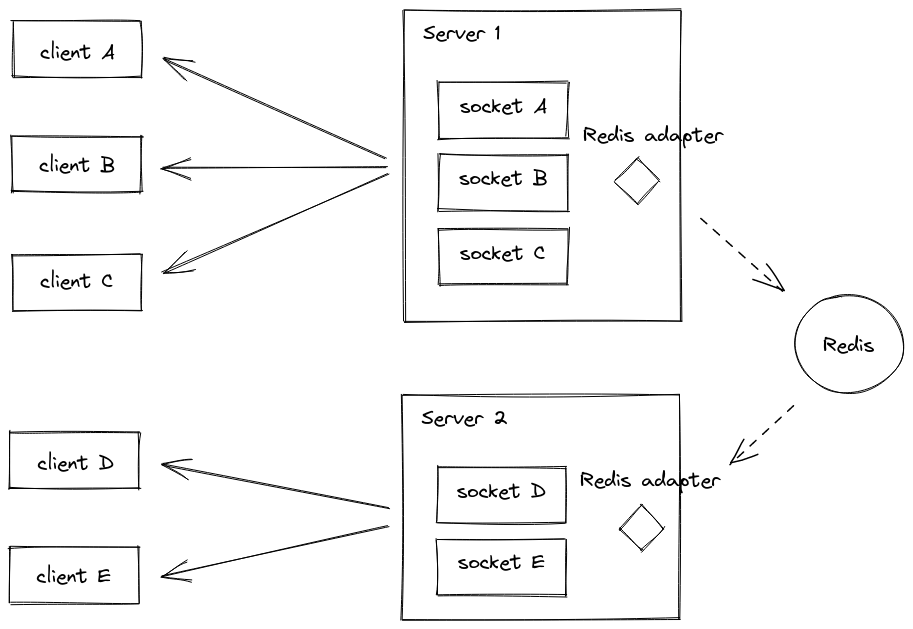
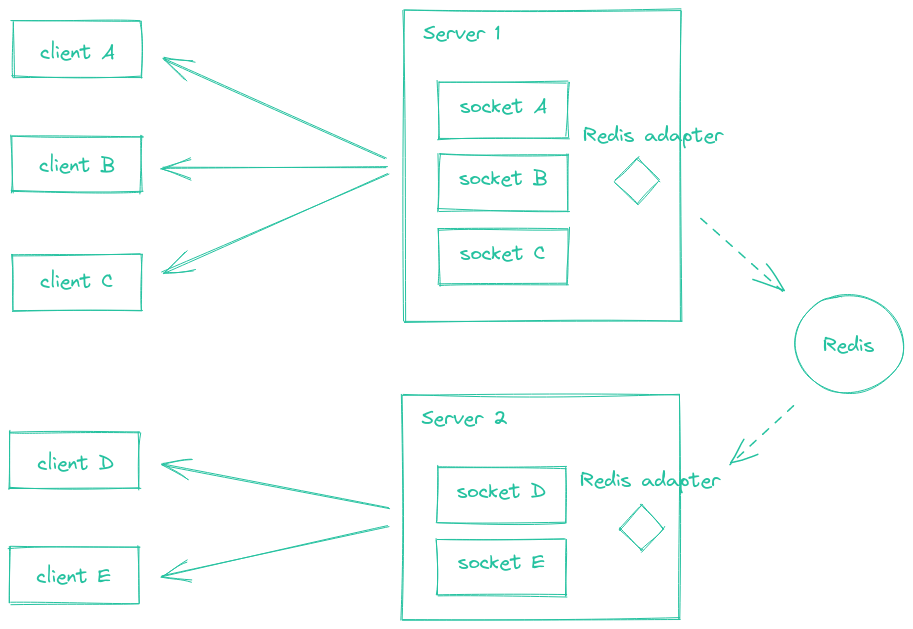
在某些情况下,你可能只想向连接到当前服务器的客户端广播。你可以使用 local
标志来实现此目的:
¥In certain cases, you may want to only broadcast to clients that are connected to the current server. You can achieve this with the local
flag:
io.local.emit("hello", "world");
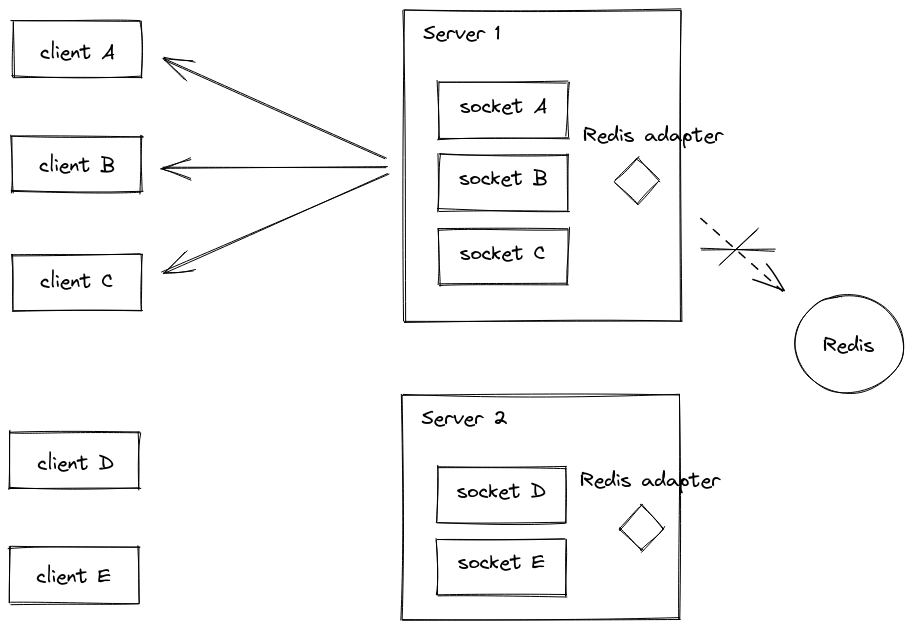
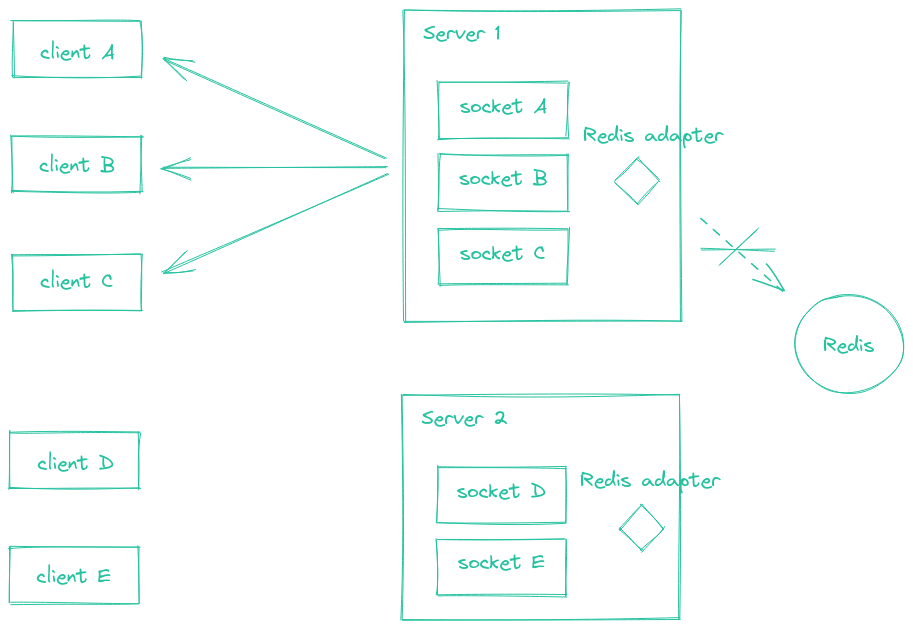
为了在广播时针对特定客户端,请参阅有关 房间 的文档。
¥In order to target specific clients when broadcasting, please see the documentation about Rooms.